Linux APUE学习:1、文件I/O操作
文件源代码:https://github.com/RoxyKko/APUE.git
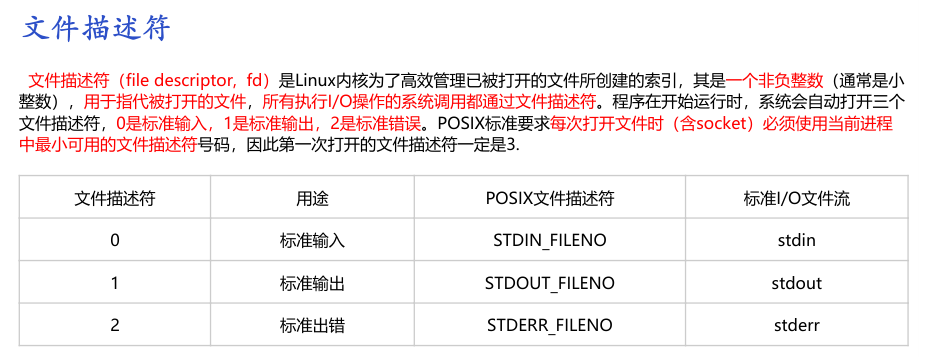
文件描述符
stdout.c
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| #include <stdio.h> #include <unistd.h> #include <string.h>
#define MSG_STR "Hello World\n"
int main(int main, char *argv[]) { printf("%s", MSG_STR); fputs(MSG_STR, stdout); write(STDOUT_FILENO, MSG_STR, strlen(MSG_STR));
return 0; }
|
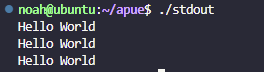
文件I/O操作
file_io.c
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68
| #include <stdio.h> #include <errno.h>
#include <unistd.h>
#include <string.h> #include <sys/types.h> #include <sys/stat.h> #include <fcntl.h>
#define BUFSIZE 1024 #define MSG_STR "Hello World\n"
int main(int argc, char *argv[]) { int fd = -1; int rv = -1; char buf[BUFSIZE];
fd = open("test.txt", O_RDWR|O_CREAT|O_TRUNC, 0666); if(fd < 0) { perror("Open/Create file test.txt failure"); return 0; } printf("Open file returned file descriptor [%d]\n", fd); if( (rv=write(fd, MSG_STR, strlen(MSG_STR))) < 0 ) { printf("Write %d bytes into failure: %s\n", rv, strerror(errno)); goto cleanup; }
lseek(fd, 0, SEEK_SET); memset(buf, 0, sizeof(buf)); if( (rv=read(fd, buf, sizeof(buf))) < 0) { printf("Read data from file failure : %s\n", strerror(errno)); goto cleanup; }
printf("Read %d bytes data from file: %s\n", rv, buf);
cleanup: close(fd); return 0; }
|


dup() 和 dup2()系统调用
常用在标准输入、标准输出、标准出错重定向。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| #include <stdio.h> #include <errno.h> #include <string.h> #include <sys/types.h> #include <sys/stat.h> #include <unistd.h> #include <fcntl.h>
int main(int argc, char *argv[]) { int fd = -1; fd = open("std.txt", O_RDWR|O_CREAT|O_TRUNC, 0666); if(fd < 0) { printf("Open file failure: %s\n", strerror(errno)); return 0; }
dup2(fd, STDIN_FILENO); dup2(fd, STDOUT_FILENO); dup2(fd, STDERR_FILENO);
printf("fd = %d\n", fd);
close(fd); return 0; }
|

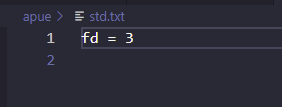
stat()和fstat()系统调用
stat()可以直接使用,fstat()要先open()后使用,两者都是系统调用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| #include <stdio.h> #include <unistd.h> #include <sys/types.h> #include <sys/stat.h> #include <fcntl.h> #include <stdlib.h>
int main(int argc, char *argv[]) { struct stat *stbuff; stbuff = malloc(sizeof(struct stat));
stat("stat.c", stbuff); printf("File Mode: %o Real Size: %luB, Space Size: %luB\n", stbuff->st_mode, stbuff->st_size, stbuff->st_blksize); return 0;
}
|

access()系统调用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| #include <stdio.h> #include <unistd.h>
#define TEST_FILE "access"
int main(int argc, char *argv[]) { if(access(TEST_FILE, F_OK) != 0) { printf("File %s not exist!\n", TEST_FILE); return 0; }
printf("File %s exist!\n", TEST_FILE); if(access(TEST_FILE, R_OK) == 0) { printf("READ OK\n"); } if(access(TEST_FILE, W_OK) == 0) { printf("WRITE OK\n"); } if(access(TEST_FILE, X_OK) == 0) { printf("EXECUTE OK\n"); } return 0; }
|